README
¶
Nirvana
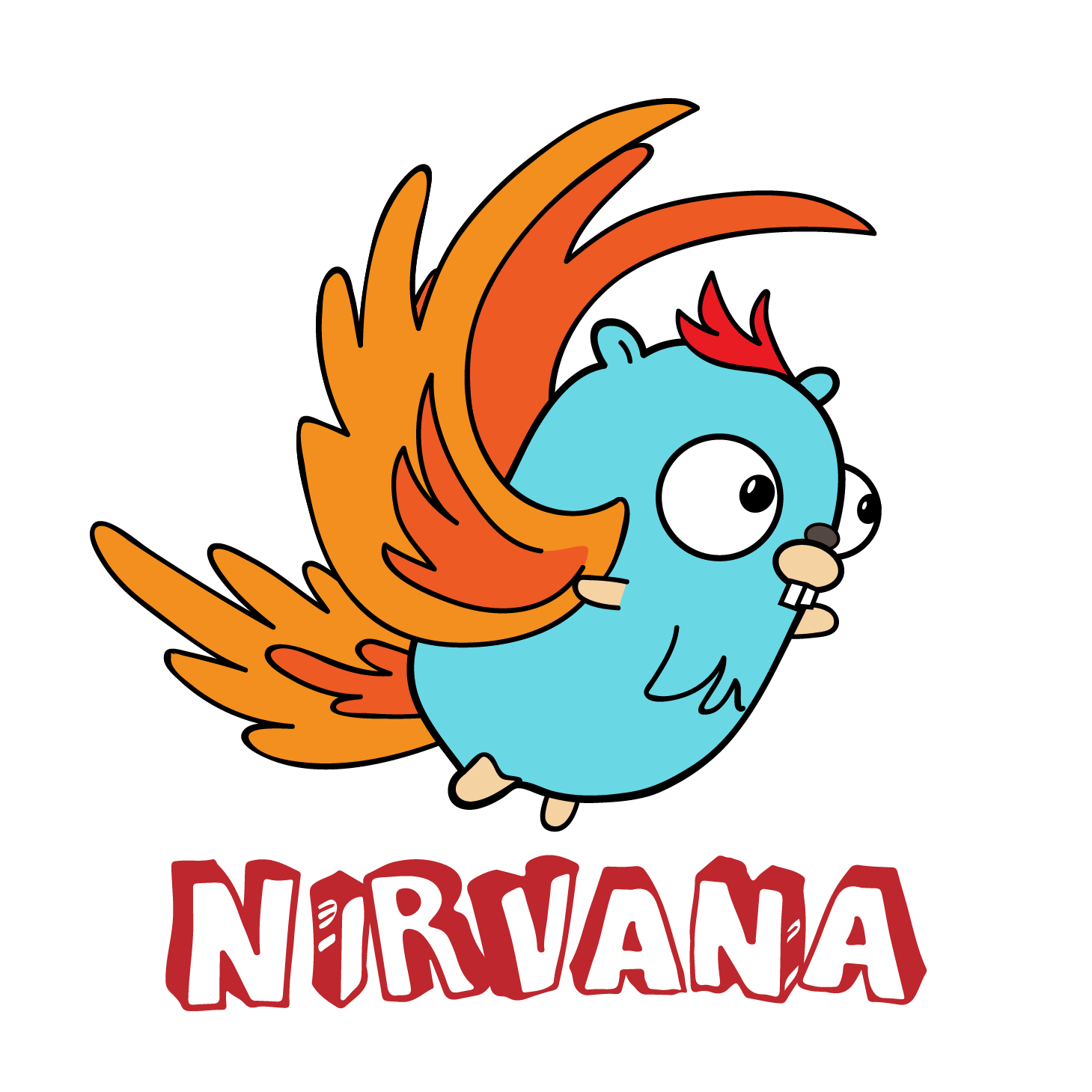
Nirvana is a golang API framework designed for productivity and usability. It aims to be the building block for all golang services at Caicloud. The high-level goals and features include:
- consistent API behavior, structure and layout across all golang projects
- improve engineering productivity with openAPI and client generation, etc
- validation can be added by declaring validation method as part of API definition
- out-of-box instrumentation support, e.g. metrics, profiling, tracing, etc
- easy and standard configuration management, as well as standard cli interface
Nirvana is also extensible and performant, with the goal to support fast developmenet velocity.
Getting Started
Nirvana provides documentations in two languages to help you expore this framework. Note right now, only Chinese docs are kept up-to-date.
Features
- API Framework based on Descriptors
- Request Filter
- Middleware
- Validator
- Plugins
- API Doc Generation
- Client Generation
Contributing
If you are interested in contributing to Nirvana, please checkout CONTRIBUTING.md. We welcome any code or non-code contribution!
Licensing
Nirvana is licensed under the Apache License, Version 2.0. See LICENSE for the full license text.
Documentation
¶
Index ¶
- Constants
- func RegisterConfigInstaller(ci ConfigInstaller)
- type Config
- type ConfigInstaller
- type Configurer
- func Descriptor(descriptors ...definition.Descriptor) Configurer
- func Filter(filters ...service.Filter) Configurer
- func IP(ip string) Configurer
- func Logger(logger log.Logger) Configurer
- func Modifier(modifiers ...service.DefinitionModifier) Configurer
- func Port(port uint16) Configurer
- func TLS(certFile, keyFile string) Configurer
- type Server
Constants ¶
const Banner = `` /* 307-byte string literal not displayed */
Banner shows an ASCII art of nirvana.
const Logo = `` /* 2299-byte string literal not displayed */
Logo presents an ASCII art of nirvana logo.
Variables ¶
This section is empty.
Functions ¶
func RegisterConfigInstaller ¶
func RegisterConfigInstaller(ci ConfigInstaller)
RegisterConfigInstaller registers a config installer.
Types ¶
type Config ¶
type Config struct {
// contains filtered or unexported fields
}
Config describes configuration of server.
func NewConfig ¶
func NewConfig() *Config
NewConfig creates a pure config. If you don't know how to set filters and modifiers for specific scenario, please use NewDefaultConfig().
func NewDefaultConfig ¶
func NewDefaultConfig() *Config
NewDefaultConfig creates default config. Default config contains:
Filters: RedirectTrailingSlash, FillLeadingSlash, ParseRequestForm. Modifiers: FirstContextParameter, ConsumeAllIfConsumesIsEmpty, ProduceAllIfProducesIsEmpty, ConsumeNoneForHTTPGet, ConsumeNoneForHTTPDelete, ProduceNoneForHTTPDelete.
func (*Config) Configure ¶
func (c *Config) Configure(configurers ...Configurer) *Config
Configure configs by configurers. It panics if an error occurs or config is locked.
type ConfigInstaller ¶
type ConfigInstaller interface { // Name is the external config name. Name() string // Install installs stuffs before server starting. Install(builder service.Builder, config *Config) error // Uninstall uninstalls stuffs after server terminating. Uninstall(builder service.Builder, config *Config) error }
ConfigInstaller is used to install config to service builder.
func ConfigInstallerFor ¶
func ConfigInstallerFor(name string) ConfigInstaller
ConfigInstallerFor gets installer by name.
type Configurer ¶
Configurer is used to configure server config.
func Descriptor ¶
func Descriptor(descriptors ...definition.Descriptor) Configurer
Descriptor returns a configurer to add descriptors into config.
func Filter ¶
func Filter(filters ...service.Filter) Configurer
Filter returns a configurer to add filters into config.
func Logger ¶
func Logger(logger log.Logger) Configurer
Logger returns a configurer to set logger into config.
func Modifier ¶
func Modifier(modifiers ...service.DefinitionModifier) Configurer
Modifier returns a configurer to add definition modifiers into config.
func TLS ¶ added in v0.2.0
func TLS(certFile, keyFile string) Configurer
TLS returns a configurer to set certFile and keyFile in config.
type Server ¶
type Server interface { // Serve starts to listen and serve requests. // The method won't return except an error occurs. Serve() error // Shutdown gracefully shuts down the server without interrupting any // active connections. Shutdown(ctx context.Context) error // Builder create a service builder for current server. Don't use this method directly except // there is a special server to hold http services. After server shutdown, clean resources via // returned cleaner. // This method always returns same builder until cleaner is called. Then it will // returns new one. Builder() (builder service.Builder, cleaner func() error, err error) }
Server is a complete API server. The server contains a router to handle all requests form clients.