Keploy
Keploy is a no-code testing platform that generates tests from API calls.
It captures the external dependency network calls (like database queries, internal/external services) for each request to replay them (including writes/mutations!) later during testing.
Developers can use keploy alongside their favorite unit testing framework to save time writing testcases.
Coolest features
- Generates test cases from API calls. Say B-Bye! to writing unit and API test cases.
- Automatically mock network/external dependencies with correct responses. No more manually writing mocks for dependencies like DBs, internal services, or third party services like twilio, shopify or stripe.
- Safely replay writes or mutations by capturing from local or other environments. Idempotency guarantees are also not required in the application. Multiple Read after write operations can be replicated automatically too.
- Statistical deduplication ensures that redundant testcases are not generated. We're planning to make this more robust (ref #27).
- Web Console to visually understand the results, update behaviour and share findings across your team.
- Native interoperability with popular testing libraries like go-test to enable compatibility with existing test cases and CI pipelines/infrastructure.
- Automatic instrumentation for popular libraries/drivers like sql, http, grpc, etc.
- Instrumentation/Integration framework to easily add the new libraries/drivers with ~100 lines of code.
How it works?
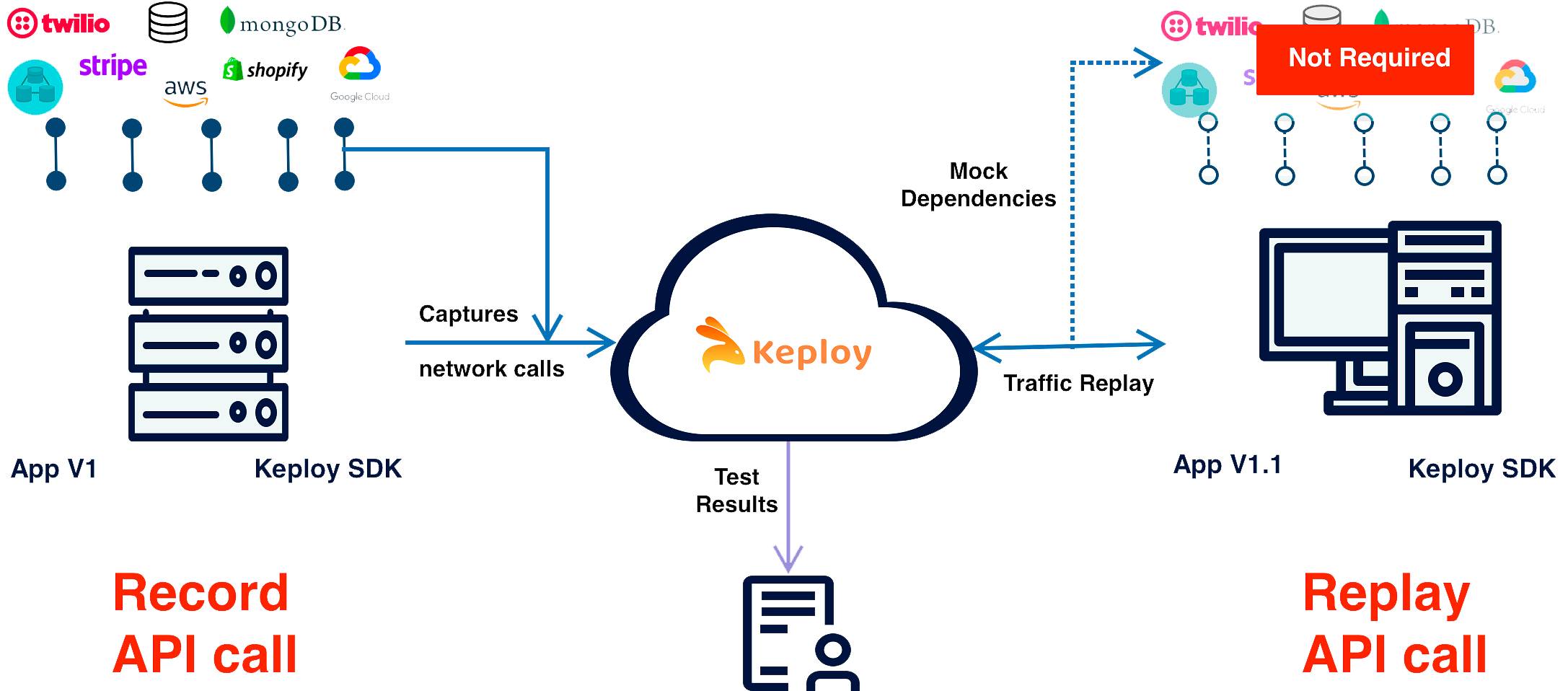
Note: You can generate test cases from any environment which has all the infrastructure dependencies setup. Please consider using this to generate tests from low-traffic environments first. The deduplication feature necessary for high-traffic environments is currently experimental.
Quickstart
Start keploy server
git clone https://github.com/keploy/keploy.git && cd keploy
docker-compose up
The UI can be accessed at http://localhost:8081
Integrate the SDK
Install the Go SDK
go get -u github.com/keploy/go-sdk
Routers
Example of integrating the gin router. Other routers like echo, chi, etc are support too.
import (
"github.com/keploy/go-sdk/integrations/kgin/v1"
"github.com/keploy/go-sdk/keploy"
)
r := gin.New()
port := "6060"
k := keploy.New(keploy.Config{
App: keploy.AppConfig{
// your application
Name: "my-app",
Port: port,
},
Server: keploy.ServerConfig{
URL: "http://localhost:8081/api",
},
})
//Call kgin.GinV1 before routes handling
kgin.GinV1(k, r)
r.Run(":" + port)
Datastore
Example of integrating the official mongo driver. Other datastore/database libraries like go's sql
import "github.com/keploy/go-sdk/integrations/kmongo"
db := client.Database("MyDB")
// wrap collection with keploy for automatic instrumentation and mocking
col := kmongo.NewMongoCollection(db.Collection("MyCollection"))
Thats it! All the requests after integration will be automatically captured and available it at http://localhost:8081/testlist
Integration with native go test framework
You just need 3 lines of code in your unit test file and that's it!!🔥🔥🔥
import (
"github.com/keploy/go-sdk/keploy"
"testing"
)
func TestKeploy(t *testing.T) {
keploy.SetTestMode()
go main()
keploy.AssertTests(t)
}
Note: You can also try a sample URL shortner application for better understanding.
Language Support
Development
There's a separate docker-compose file which helps which exposes the mongo server and also remote debugging port. The build
flag ensures that the binary is built again to reflect the latest code changes.
docker-compose -f docker-compose-dev.yaml up --build
If you are not using docker, you can build and run the keploy server directly. Ensure to provide the Mongo connection string via the KEPLOY_MONGO_URI
env variable.
export KEPLOY_MONGO_URI="mongodb://mongo:27017"
go run cmd/server/main.go
Keploy exposes GraphQL API for the frontend based on gqlgen. After changing the schema you can autogenerate graphQL handlers using
go generate ./...
We'd love to collaborate with you to make Keploy great. To get started:
- Slack - Discussions with the community and the team.
- GitHub - For bug reports and feature requests.