Testcontainers-Go Common Image Registry

Common Image Registry for Testcontainers-Go
Prerequisites
Install
go get go.nhat.io/testcontainers-registry
Elasticsearch
package example
import (
"context"
es "go.nhat.io/testcontainers-registry/elasticsearch"
testcontainers "go.nhat.io/testcontainers-extra"
)
const (
dbName = "test"
migrationSource = "file://./resources/migrations/"
)
func startElasticsearch() (testcontainers.Container, error) {
return es.StartGenericContainer(context.Background())
}
Mongo
package example
import (
"context"
"go.nhat.io/testcontainers-registry/mongo"
testcontainers "go.nhat.io/testcontainers-extra"
)
const (
dbName = "test"
migrationSource = "file://./resources/migrations/"
)
func startMongoDB() (testcontainers.Container, error) {
return mongo.StartGenericContainer(context.Background(),
mongo.RunMigrations(migrationSource, dbName),
)
}
MySQL
package example
import (
"context"
"go.nhat.io/testcontainers-registry/mysql"
testcontainers "go.nhat.io/testcontainers-extra"
)
const (
dbName = "test"
dbUser = "test"
dbPassword = "test"
migrationSource = "file://./resources/migrations/"
)
func startMySQL() (testcontainers.Container, error) {
return mysql.StartGenericContainer(context.Background(),
dbName, dbUser, dbPassword,
mysql.RunMigrations(migrationSource),
)
}
Postgres
package example
import (
"context"
"go.nhat.io/testcontainers-registry/postgres"
testcontainers "go.nhat.io/testcontainers-extra"
)
const (
dbName = "test"
dbUser = "test"
dbPassword = "test"
migrationSource = "file://./resources/migrations/"
)
func startPostgres() (testcontainers.Container, error) {
return postgres.StartGenericContainer(context.Background(),
dbName, dbUser, dbPassword,
postgres.RunMigrations(migrationSource),
)
}
SQL Server
package example
import (
"context"
testcontainers "go.nhat.io/testcontainers-extra"
"go.nhat.io/testcontainers-registry/mssql"
)
const (
dbName = "test"
dbPassword = "My!StrongPassword"
migrationSource = "file://./resources/migrations/"
)
func startSQLServer() (testcontainers.Container, error) {
return mssql.StartGenericContainer(context.Background(),
dbName, dbPassword,
mssql.RunMigrations(migrationSource),
)
}
Options
Change Image Tag
package example
import (
"context"
"go.nhat.io/testcontainers-registry/postgres"
testcontainers "go.nhat.io/testcontainers-extra"
)
const (
dbName = "test"
dbUser = "test"
dbPassword = "test"
)
func startPostgres() (testcontainers.Container, error) {
return postgres.StartGenericContainer(context.Background(),
dbName, dbUser, dbPassword,
testcontainers.WithImageTag("13-alpine"),
)
}
Change Image Name
package example
import (
"context"
testcontainers "go.nhat.io/testcontainers-extra"
"go.nhat.io/testcontainers-registry/mysql"
)
const (
dbName = "test"
dbUser = "test"
dbPassword = "test"
)
func startMySQL() (testcontainers.Container, error) {
return mysql.StartGenericContainer(context.Background(),
dbName, dbUser, dbPassword,
testcontainers.WithImageName("mariadb"),
testcontainers.WithImageTag("10.7"),
)
}
Donation
If this project help you reduce time to develop, you can give me a cup of coffee :)
Paypal donation
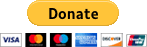
or scan this